MATLAB is a powerful programming language and environment widely used in various fields, including engineering, science, and data analysis. Whether you're a beginner or an experienced programmer, these essential programming tips will help you master MATLAB and boost your productivity.
1. Understand the Basics

Before diving into advanced techniques, ensure you have a solid grasp of the fundamentals. Learn the syntax, data types, variables, and basic operations in MATLAB. Familiarize yourself with the MATLAB workspace, command window, and editor.
- Data Types: MATLAB supports various data types such as integers, doubles, characters, and logical values. Understand how to declare and manipulate these data types effectively.
- Variables: Learn how to assign values to variables and access them throughout your code. MATLAB allows for dynamic typing, so you don't need to explicitly declare variable types.
- Operators: Get familiar with MATLAB's arithmetic, relational, and logical operators. These operators are essential for performing calculations and comparisons in your code.
2. Efficient Array Operations
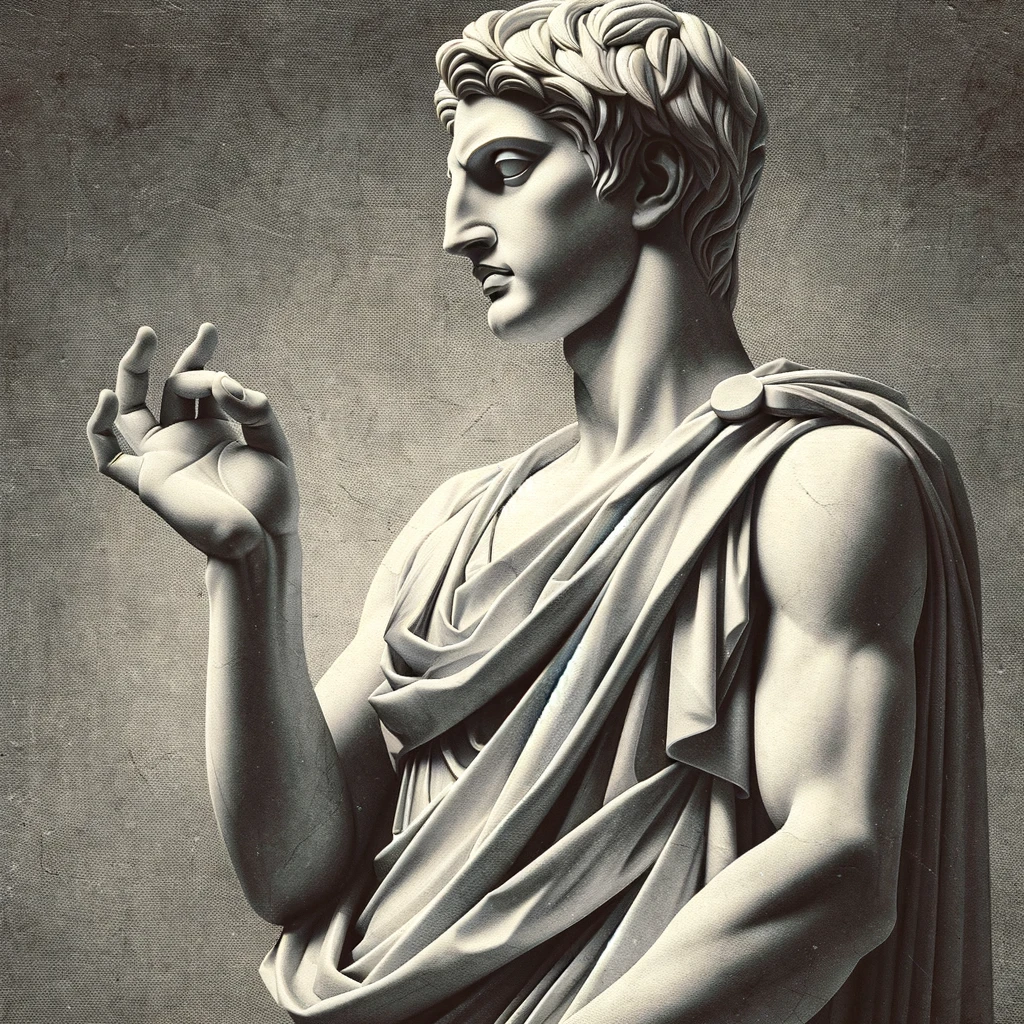
MATLAB is renowned for its array-based operations. Optimize your code by utilizing built-in functions and matrix operations.
- Matrix Operations: Leverage MATLAB's matrix capabilities to perform efficient calculations. Functions like
sum
,mean
,max
, andmin
can be applied to entire arrays, making your code more concise and readable. - Array Indexing: Learn how to access and manipulate specific elements of arrays using indexing techniques. MATLAB allows for advanced indexing methods, such as linear indexing and logical indexing, to efficiently access and modify array data.
- Built-in Functions: MATLAB provides a vast collection of built-in functions for array operations. Familiarize yourself with functions like
find
,unique
,sort
, anddiff
to simplify your code and improve performance.
3. Utilize MATLAB's Powerful Toolboxes
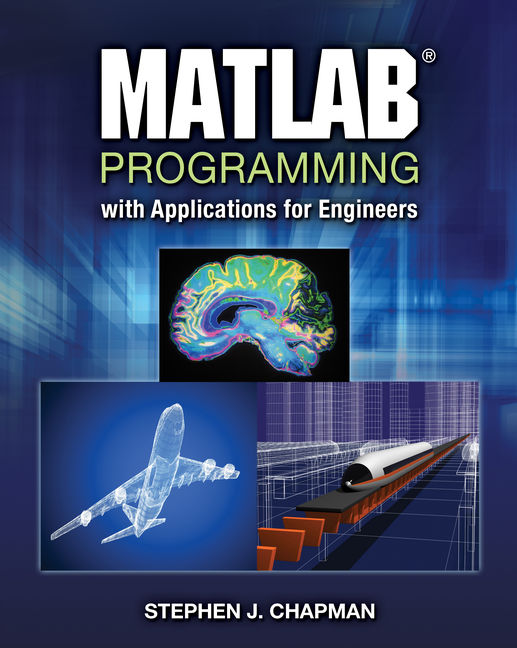
MATLAB offers a wide range of toolboxes that extend its functionality in various domains. Explore and utilize these toolboxes to enhance your programming capabilities.
- Signal Processing Toolbox: If you work with signals and systems, this toolbox provides functions for filtering, spectrum analysis, and signal generation.
- Image Processing Toolbox: For image analysis and manipulation tasks, this toolbox offers a comprehensive set of functions for image processing and computer vision.
- Statistics and Machine Learning Toolbox: If you're involved in data analysis or machine learning, this toolbox provides functions for statistical analysis, regression, classification, and clustering.
- Optimization Toolbox: This toolbox is invaluable for optimization problems, offering algorithms for nonlinear optimization, linear programming, and constrained optimization.
4. Efficient Memory Management

Efficient memory management is crucial for optimizing your MATLAB code. Large datasets or complex computations can consume significant memory, impacting performance.
- Avoid Large Temporary Arrays: Minimize the creation of large temporary arrays that are not necessary for your calculations. Instead, use in-place operations or preallocate memory to improve performance.
- Clear Unnecessary Variables: Regularly clear variables that are no longer needed in your workspace. This frees up memory and prevents unnecessary memory usage.
- Use Persistent Variables: Persistent variables retain their values between function calls, reducing the need to reallocate memory. Use them wisely to optimize memory usage.
5. Optimize Loop Performance

Loops are essential for repetitive tasks, but they can impact performance. Optimize your loops to improve execution speed.
- Vectorize Your Code: Instead of using explicit loops, utilize vectorized operations whenever possible. Vectorization allows MATLAB to perform operations on entire arrays, leading to significant performance improvements.
- Use Built-in Functions: Many built-in functions in MATLAB are optimized for speed. Prefer using these functions over custom loop implementations for common operations.
- Loop Unrolling: Consider loop unrolling to reduce function call overhead. By repeating the loop body multiple times, you can eliminate the overhead of function calls, improving performance.
6. Effective Debugging Techniques
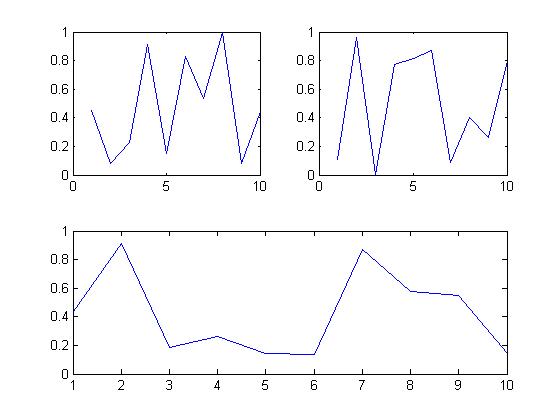
Debugging is an essential skill for any programmer. MATLAB provides various tools and techniques to help you identify and fix errors in your code.
- Use the MATLAB Debugger: The built-in MATLAB debugger allows you to step through your code, inspect variables, and set breakpoints. Utilize this tool to identify and resolve issues effectively.
- Error Messages and Warnings: Pay attention to error messages and warnings generated by MATLAB. These messages provide valuable information about the nature of the problem and can guide you towards a solution.
- Assert Statements: Use assert statements to verify conditions and catch errors early in your code. Assert statements can help you identify unexpected behavior and ensure the correctness of your program.
7. Efficient File I/O
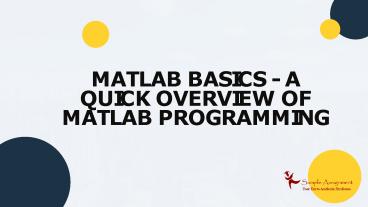
Efficient file input and output operations are crucial for working with large datasets or saving and loading data.
- Use Built-in Functions: MATLAB provides a wide range of functions for reading and writing files, such as
fopen
,fread
,fwrite
, andfclose
. Familiarize yourself with these functions to streamline your file I/O operations. - Consider Memory-Mapped Files: For large datasets, memory-mapped files can be an efficient alternative to traditional file I/O. This approach allows you to access files directly from memory, reducing the overhead of copying data.
- Use Binary Format: When working with large datasets, consider using binary format for data storage. Binary files are more compact and can be read and written faster compared to text-based formats.
8. Parallel Computing
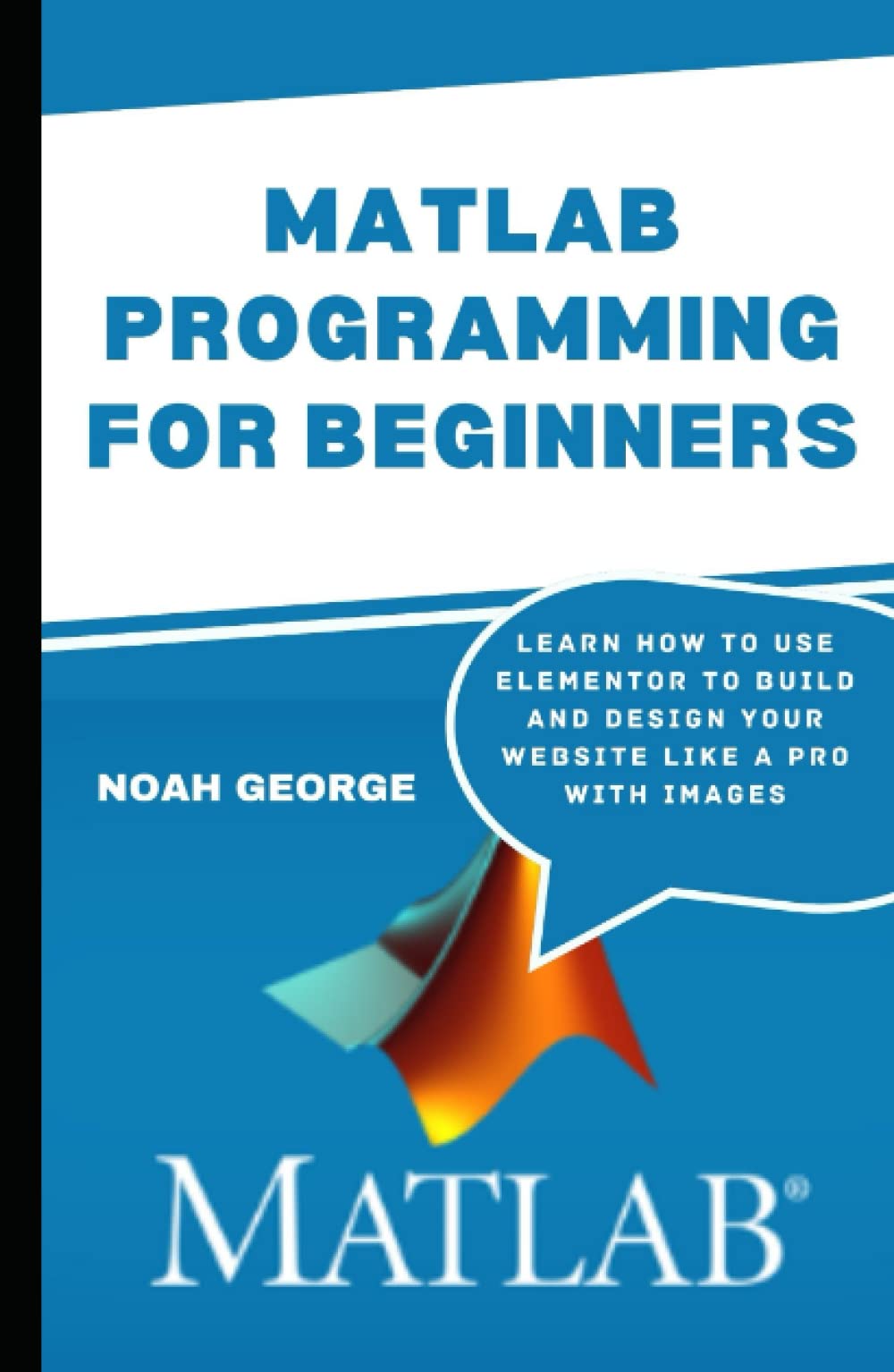
MATLAB supports parallel computing, allowing you to leverage multiple processors or cores to speed up your computations.
- Parallel Computing Toolbox: MATLAB's Parallel Computing Toolbox provides functions and tools for parallelizing your code. Explore this toolbox to take advantage of parallel processing and improve performance.
- Parallel For-Loops: Use parallel for-loops to distribute loop iterations across multiple processors or cores. This can significantly speed up computations involving repetitive tasks.
- Distributed Computing: If you have access to a cluster of computers, you can utilize distributed computing to run your MATLAB code across multiple machines, further enhancing performance.
9. Code Documentation and Comments
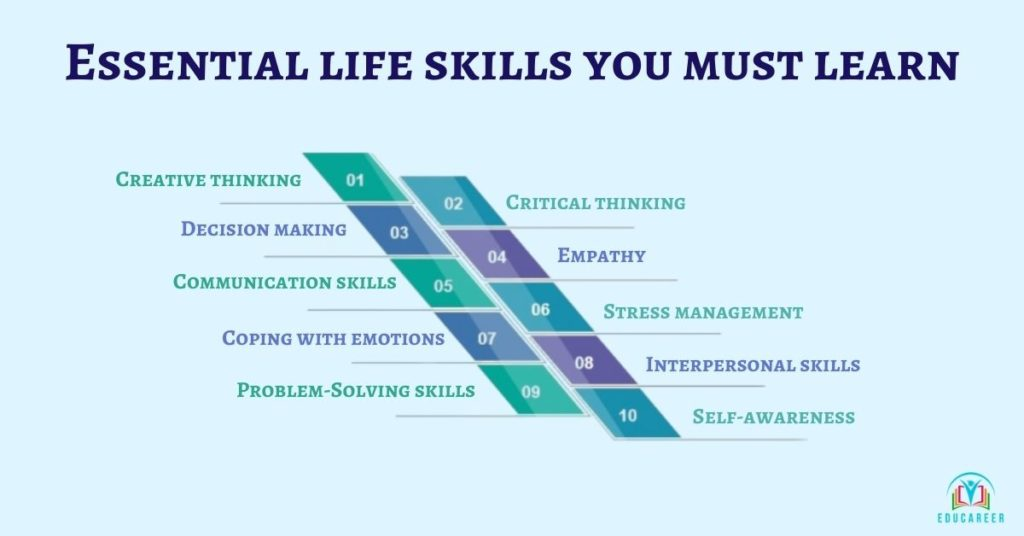
Writing well-documented code is essential for maintaining and sharing your MATLAB programs. Clear and concise comments improve code readability and help others (or your future self) understand your code.
- Comment Your Code: Add comments to explain the purpose of functions, variables, and complex sections of code. Use comments to provide context and make your code more self-explanatory.
- Use MATLAB's Built-in Documentation: MATLAB provides extensive documentation for its functions and toolboxes. Familiarize yourself with this documentation to understand the available functions and their usage.
- Write Clear Function Names and Descriptions: Choose descriptive function names and provide clear function descriptions to make your code more accessible and understandable.
10. Regularly Update and Learn
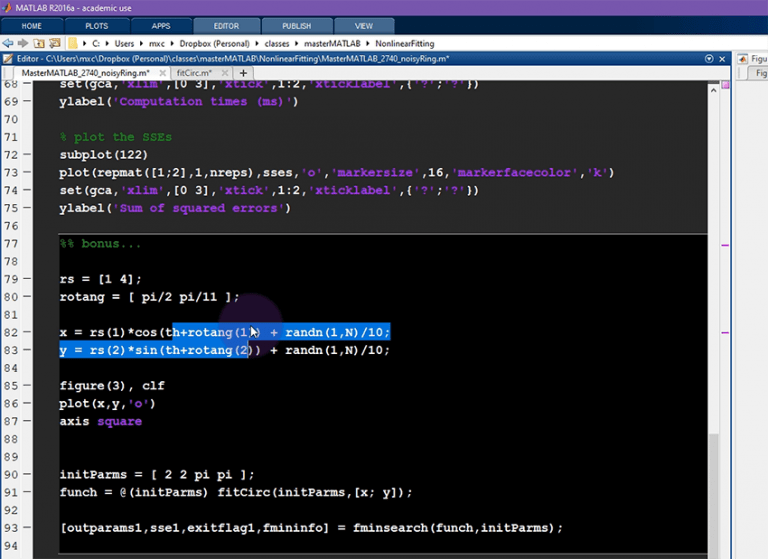
MATLAB is constantly evolving, with new features and improvements being introduced regularly. Stay updated with the latest versions and learn from the MATLAB community.
- Keep MATLAB Updated: Regularly update your MATLAB installation to access the latest features and bug fixes. MATLAB's automatic update feature makes it easy to stay up-to-date.
- Explore Online Resources: There are numerous online resources, tutorials, and forums dedicated to MATLAB programming. Explore these resources to learn new techniques, best practices, and tips from the MATLAB community.
- Join MATLAB User Groups: Engage with the MATLAB community by joining user groups or attending MATLAB events. These communities provide valuable insights, support, and opportunities to learn from experienced MATLAB users.
By following these essential programming tips, you'll be well on your way to mastering MATLAB and writing efficient, powerful code. Remember, practice makes perfect, so keep coding and exploring the vast capabilities of MATLAB.
What are some common uses of MATLAB in real-world applications?
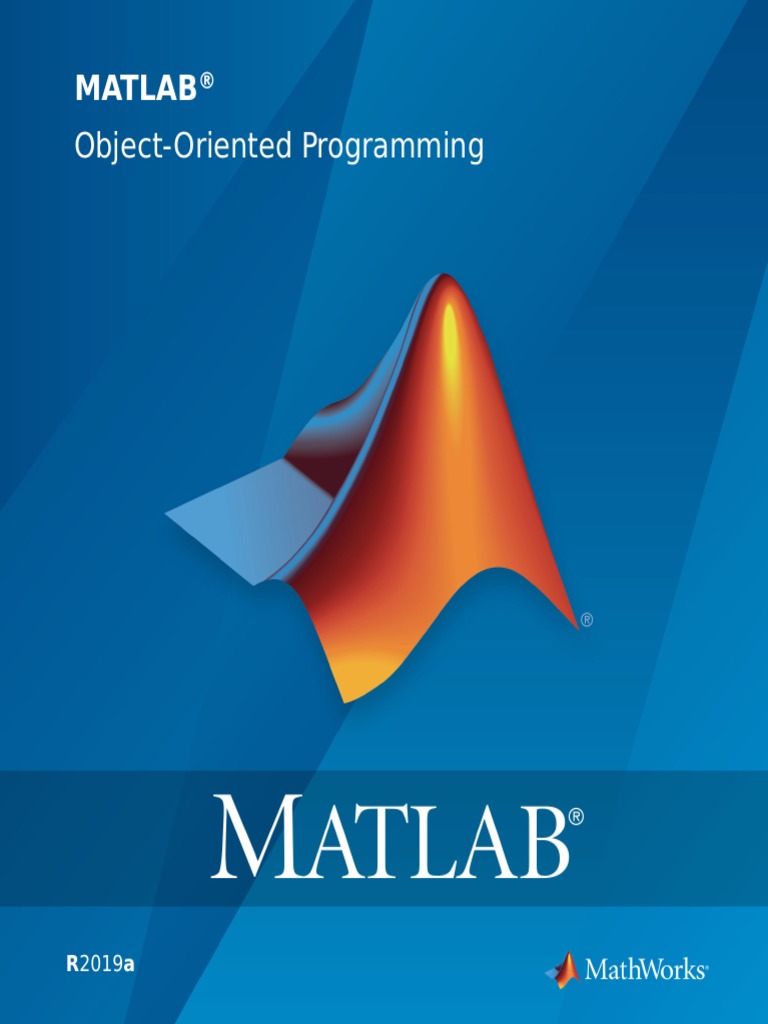
+
MATLAB is widely used in various fields, including engineering, science, finance, and machine learning. It is commonly used for data analysis, signal processing, image processing, control systems, and much more.
Can I use MATLAB for web development or mobile app development?
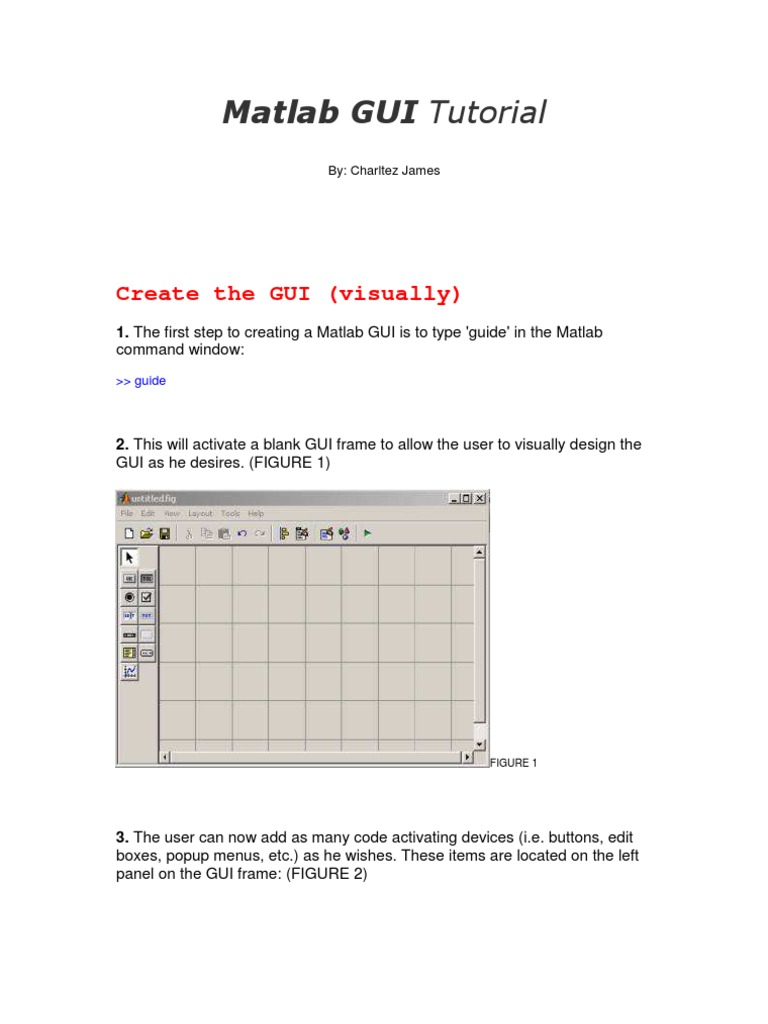
+
While MATLAB is primarily designed for numerical computing and data analysis, it can be integrated with other technologies for web or mobile development. MATLAB provides APIs and toolboxes for interfacing with web services and mobile platforms.
Are there any alternatives to MATLAB for scientific computing and data analysis?
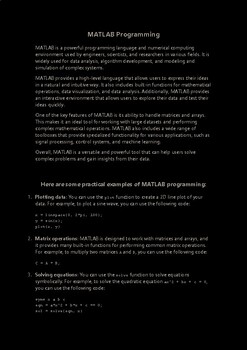
+
Yes, there are several alternatives to MATLAB, such as Python (with libraries like NumPy and SciPy), R, and Julia. These languages offer similar capabilities for scientific computing and data analysis and often have a more extensive community and open-source ecosystem.
How can I learn more advanced MATLAB programming techniques?
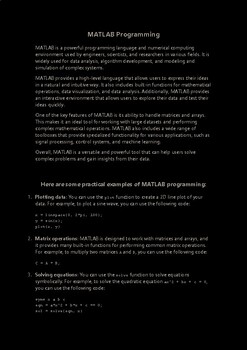
+
To enhance your MATLAB programming skills, consider exploring advanced topics such as object-oriented programming, GUI development, and optimizing code for large-scale computations. MATLAB’s documentation and online resources provide extensive tutorials and examples to guide you.